以勇气面对人生的巨大悲恸,用耐心对待生活的小小哀伤。——雨果
首先安装
1 2 3 4 5 6 7 8
| # axios cnpm i --save axios # 格式化参数插件 cnpm i -- save qs # 对象合并插件 cnpm i -- save lodash # cookie操作 cnpm i -- save vue-cookie
|

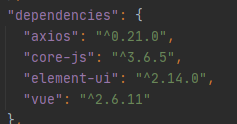
然后我们自己封装一个请求组件
首先创建文件
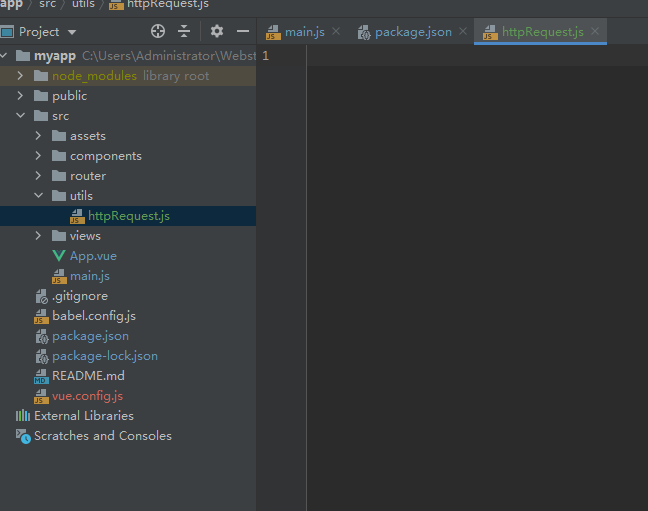
然后放入我们的代码。。。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132
| import axios from 'axios' import qs from 'qs' import merge from 'lodash/merge' import {Loading, Message} from 'element-ui' import VueCookie from 'vue-cookie';
const BASE_URL = process.env.NODE_ENV === 'production' ? process.env.VUE_APP_SERVER_URL : process.env.VUE_APP_BASE_API
axios.defaults.withCredentials = true
const http = axios.create({ timeout: 1000 * 30, withCredentials: true, headers: { 'Content-Type': 'application/json; charset=utf-8' }, notUseDefaultApi: false })
let loading http.interceptors.request.use(config => { let showLoading = false if (config.loading === true) { showLoading = true } if (showLoading) { loading = Loading.service({ text: config.loadingText || 'ruben正在全力为您加载中...', spinner: 'el-icon-loading', background: 'rgba(0, 0, 0, 0.7)' }) } config.headers.token = VueCookie.get('token') if (!config.notUseDefaultApi) { config.url = BASE_URL + config.url; } const method = config.method const params = {} const arrayFormat = config.headers.arrayFormat || 'indices' if (method === 'post' && config.headers['Content-Type'] === 'application/x-www-form-urlencoded; charset=utf-8') { config.data = qs.stringify(config.data, { allowDots: true, arrayFormat: arrayFormat }) } else if (method === 'get') { config.params = qs.stringify(config.params, { allowDots: true, arrayFormat: arrayFormat }) config.params = qs.parse(config.params) config.params = merge(params, config.params) } console.log(config) return config }, error => { return Promise.reject(error) })
let errorMessageAlertOption = { message: '请求错误', type: 'error', showClose: true, dangerouslyUseHTMLString: true, duration: 3000, customClass: 'el-icon-lightning' }
http.interceptors.response.use(response => { if (loading) { loading.close(); } if (response.data && response.data.success === false) { errorMessageAlertOption.message = response.data.msg Message(errorMessageAlertOption); } return response }, error => { if (loading) { loading.close(); } console.log(error) Message(errorMessageAlertOption) return Promise.reject(error) })
export default http
|
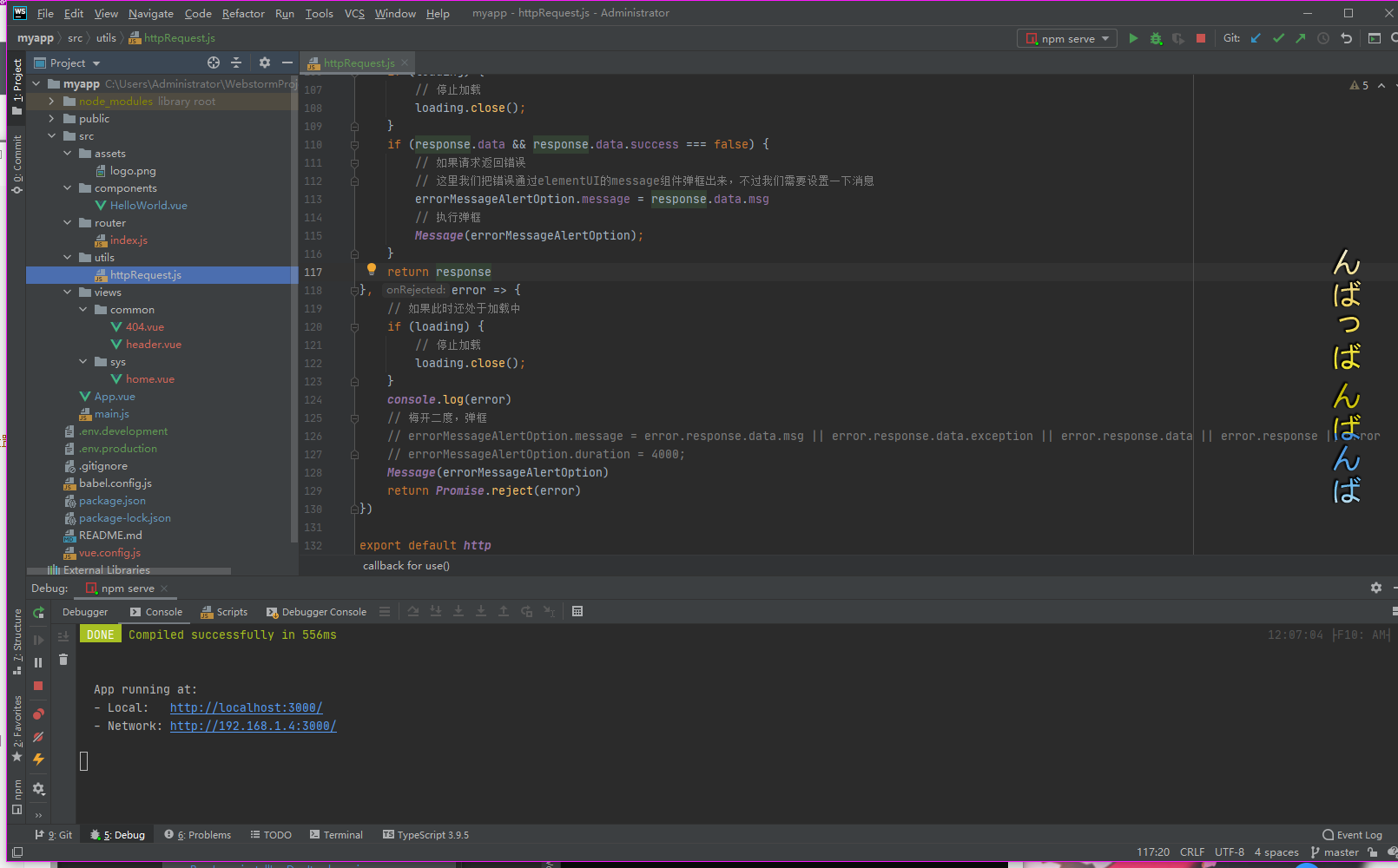
然后在main.js
引用
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| import Vue from 'vue' import App from './App.vue' import router from '@/router' import ElementUI from 'element-ui'; import 'element-ui/lib/theme-chalk/index.css'; import httpRequest from '@/utils/httpRequest' import VueCookie from 'vue-cookie';
Vue.use(ElementUI, httpRequest, VueCookie); Vue.config.productionTip = false
Vue.prototype.$http = httpRequest Vue.prototype.$cookie = VueCookie new Vue({ router, render: h => h(App), }).$mount('#app')
|
这里加了两个配置文件,别忘了
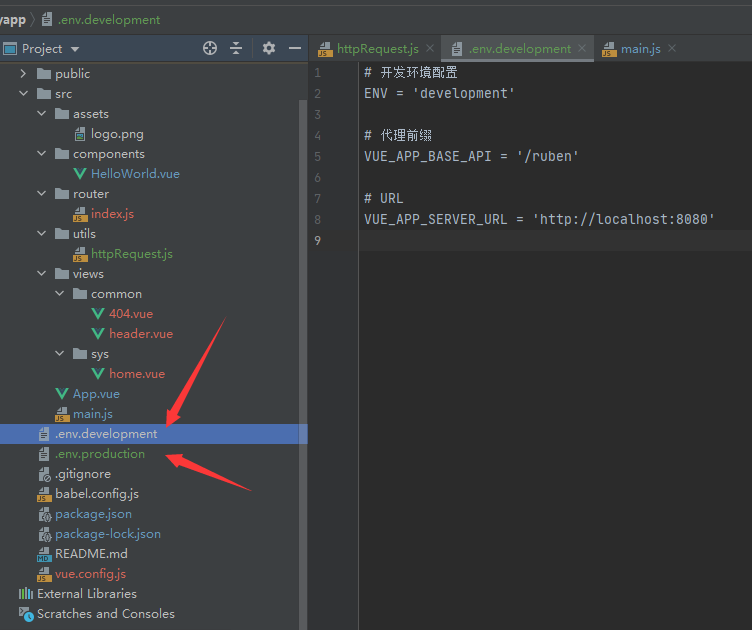
1 2 3 4 5 6 7 8
| # 开发环境配置 ENV = 'development'
# 代理前缀 VUE_APP_BASE_API = '/ruben'
# URL VUE_APP_SERVER_URL = 'http://localhost:8080'
|
以及
1 2 3 4
| # 生产环境配置 ENV = 'production'
VUE_APP_SERVER_URL = 'localhost:8080'
|
还有在vue的vue.config.js
配置文件中配置
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| module.exports = { devServer: { port: 3000, disableHostCheck: true, proxy: { '/ruben': { target: process.env.VUE_APP_SERVER_URL, changeOrigin: true, pathRewrite: { '^/ruben': '' } } } }
}
|
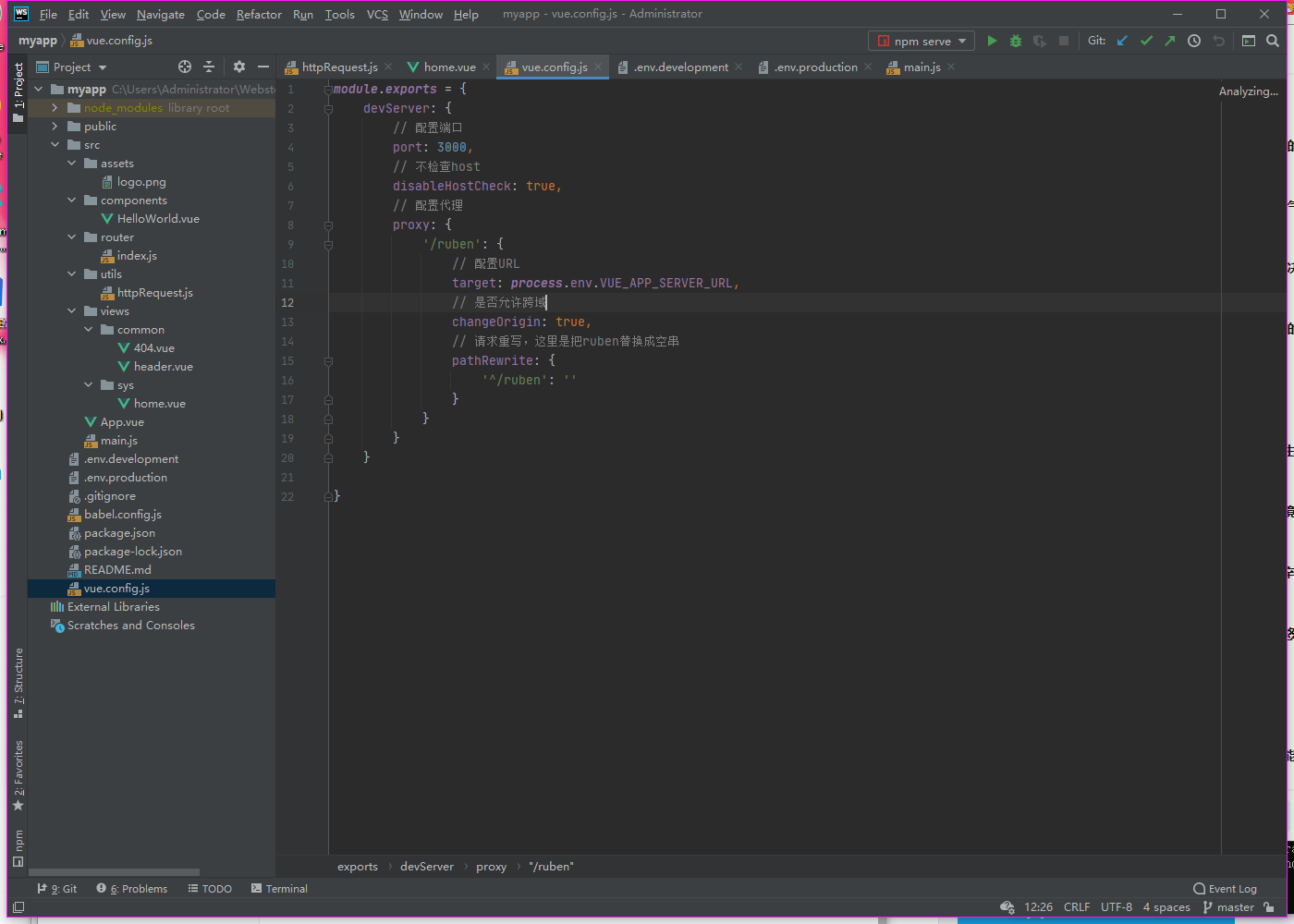
最后是使用
1 2 3 4 5 6 7 8 9 10
| this.$http({ url: `/user/login`, method: 'post', data: this.inputForm }).then(({data}) => { if (data && data.success) { this.$message.success(data.msg) console.log(data) } });
|
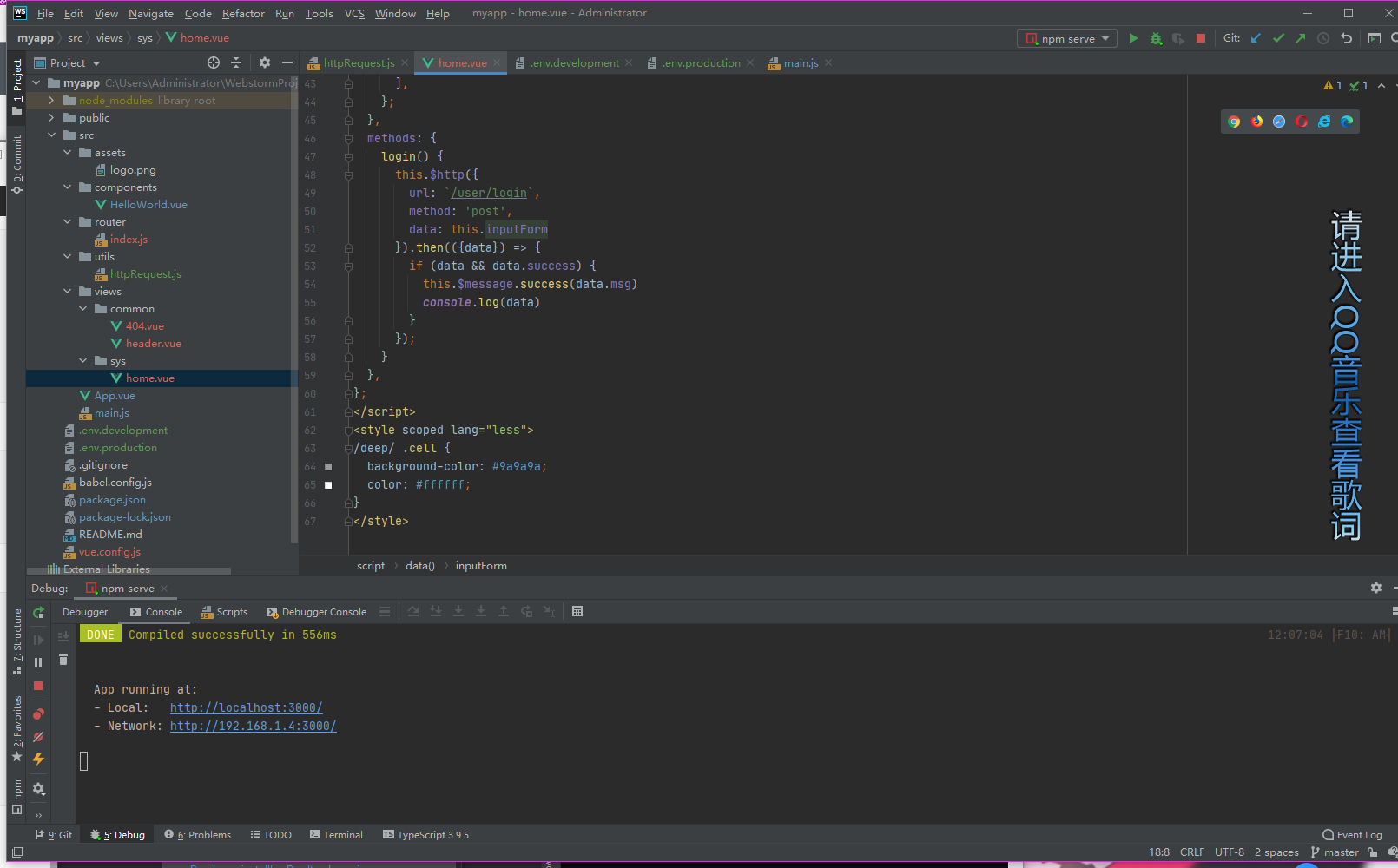
完整代码放到了gitee
仓库
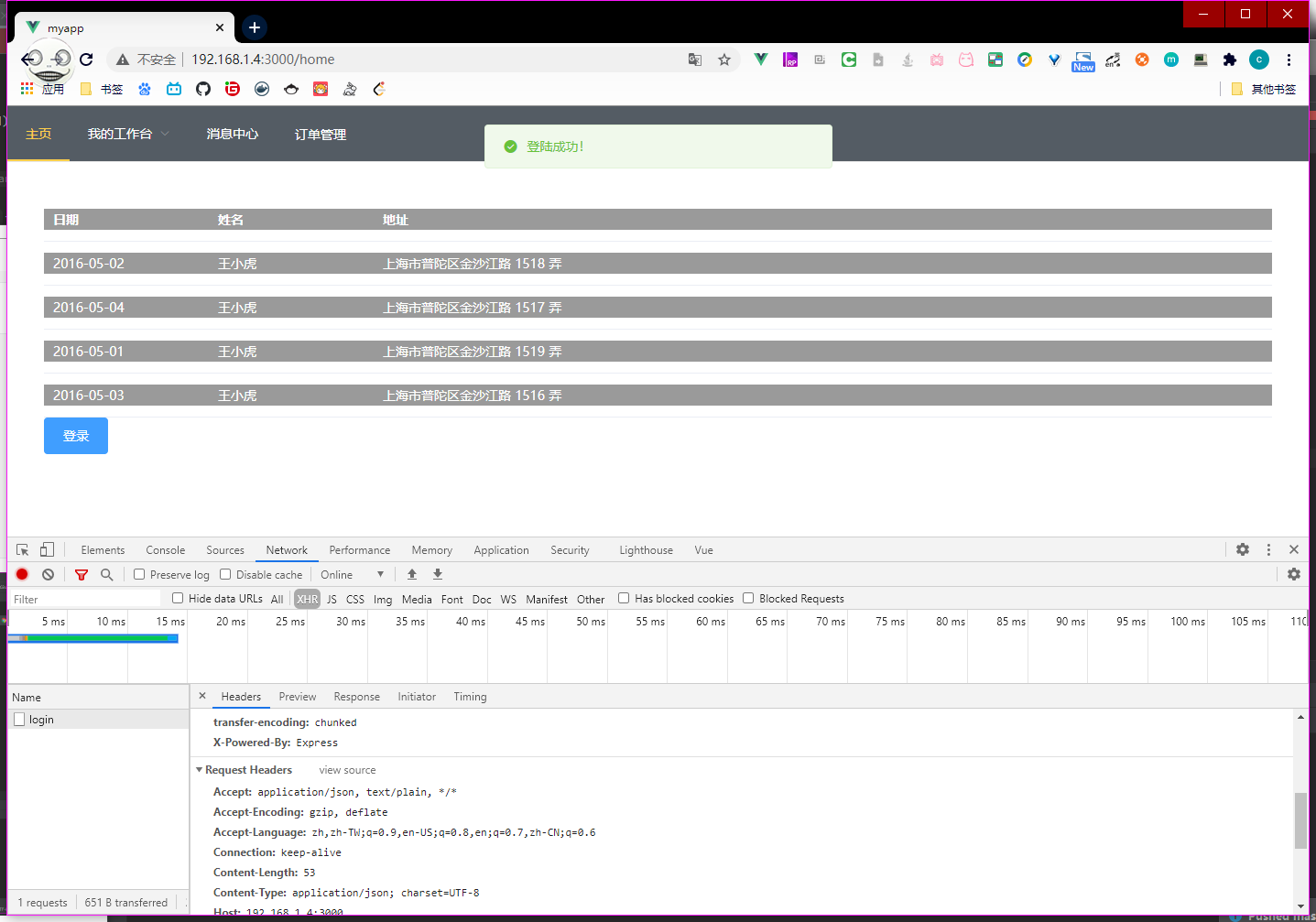
我会继续完善下去的~