如果你不出去走走,你就会以为这就是全世界。——《天堂电影院》
如果你不出去走走,你就会以为这就是全世界。——《天堂电影院》
之前写过一篇js
时间戳格式化以及一篇js
中Date
函数的api
今天写篇“人性化”的格式化的
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89
| function formatTime(time) { if (typeof time !== 'number' || time < 0) { return time }
var hour = parseInt(time / 3600) time = time % 3600 var minute = parseInt(time / 60) time = time % 60 var second = time
return ([hour, minute, second]).map(function(n) { n = n.toString() return n[1] ? n : '0' + n }).join(':') }
function formatLocation(longitude, latitude) { if (typeof longitude === 'string' && typeof latitude === 'string') { longitude = parseFloat(longitude) latitude = parseFloat(latitude) }
longitude = longitude.toFixed(2) latitude = latitude.toFixed(2)
return { longitude: longitude.toString().split('.'), latitude: latitude.toString().split('.') } }
var dateUtils = { UNITS: { '年': 31557600000, '月': 2629800000, '天': 86400000, '小时': 3600000, '分钟': 60000, '秒': 1000 }, humanize: function(milliseconds) { var humanize = ''; for (var key in this.UNITS) { if (milliseconds >= this.UNITS[key]) { humanize = Math.floor(milliseconds / this.UNITS[key]) + key + '前'; break; } } return humanize || '刚刚'; }, formatHumanize: function(dateStr) { var date = this.parse(dateStr) var diff = Date.now() - date.getTime(); if (diff < this.UNITS['天']) { return this.humanize(diff); } return this.format(date) }, parse: function(str) { var a = str.split(/[^0-9]/); return new Date(a[0], a[1] - 1, a[2], a[3], a[4], a[5]); }, format: function(date) { var _format = function(number) { return (number < 10 ? ('0' + number) : number); }; return date.getFullYear() + '-' + _format(date.getMonth() + 1) + '-' + _format(date.getDate()) + ' ' + _format(date.getHours()) + ':' + _format(date.getMinutes() + ':' + _format(date.getSeconds())); } };
console.log("秒表:" + formatTime(100)) var locationData = formatLocation(116.397128, 39.916527) console.log("经度:" + locationData.longitude) console.log("纬度:" + locationData.latitude) console.log("人性化:" + dateUtils.humanize(Date.now() - 1516026842000)) console.log("一天内人性化,一天前格式化:" + dateUtils.formatHumanize("2021-04-14 22:30:22")) console.log("一天内人性化,一天前格式化:" + dateUtils.formatHumanize("2021-04-15 22:00:22")) console.log("转换为Date对象:" + dateUtils.parse("2021-04-15 22:15:22")) console.log("格式化:" + dateUtils.format(new Date(1623766982000)))
|
打印结果
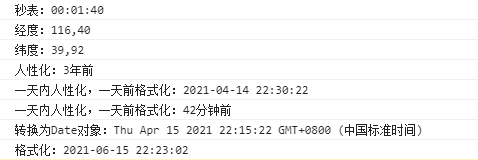